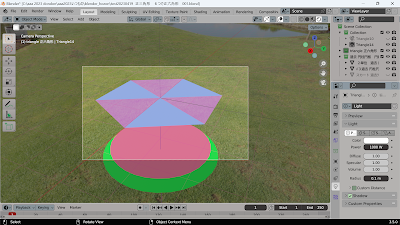
render
# コレクションを作成
import bpy
collection_name = "triangle 正六角形"
if collection_name not in bpy.data.collections:
zionad_collection = bpy.data.collections.new(collection_name)
bpy.context.scene.collection.children.link(zionad_collection)
else:
zionad_collection = bpy.data.collections[collection_name]
# コレクションを作成
import bpy
collection_name = "過去 円柱円板 円錐"
if collection_name not in bpy.data.collections:
zionad_collection = bpy.data.collections.new(collection_name)
bpy.context.scene.collection.children.link(zionad_collection)
else:
zionad_collection = bpy.data.collections[collection_name]
triangle 作ると
オブジェクト名が すべて 変更されてる
import bpy
import math
import mathutils
# 三角形の1辺の長さを指定する
triangle_size = 2.0
kakodoai = triangle_size
# 正六角形の中心位置を指定する
hexagon_center = mathutils.Vector((0.0, 0.0, 0.0))
# 6つの正三角形を作成する
for i in range(6):
# 三角形の頂点を計算する
vertex1 = mathutils.Vector((math.cos(i * math.pi / 3), math.sin(i * math.pi / 3), 0.0))
vertex2 = mathutils.Vector((math.cos((i + 1) * math.pi / 3), math.sin((i + 1) * math.pi / 3), 0.0))
vertex3 = mathutils.Vector((0.0, 0.0, 0.0))
# 三角形の頂点をサイズに合わせて調整する
vertex1 *= triangle_size
vertex2 *= triangle_size
vertex3 *= triangle_size
# 三角形のメッシュを作成する
mesh = bpy.data.meshes.new("Triangle{}".format(i+1))
mesh.from_pydata([vertex1, vertex2, vertex3], [], [(0, 1, 2)])
mesh.update()
# 三角形のオブジェクトを作成する
obj = bpy.data.objects.new("Triangle{}".format(i+1), mesh)
bpy.context.scene.collection.objects.link(obj)
# 正六角形の中心にオブジェクトを移動する
for obj in bpy.data.objects:
obj.location += hexagon_center
# オブジェクトに名前を付ける
for i, obj in enumerate(bpy.data.objects):
obj.name = "Triangle{}".format(i+1)
# 過去光円錐 (ensui_top + ensui_bottom) / 2.0)
import bpy
import math
import mathutils
# 円錐のパラメータを指定する
height = 2 #triangle_size # 2点間の距離
radius = height # 半径
ensui_top = 0 # 上部座標のz値
ensui_bottom = -2.0 #- triangle_size # 下部座標のz値
center = mathutils.Vector((0.0, 0.0, (ensui_top + ensui_bottom) / 2.0)) # 中心位置
cone_name = "スカート 過去光円錐" # オブジェクト名
# 円錐のメッシュを作成する
bpy.ops.mesh.primitive_cone_add(radius1=radius, radius2=0.0, depth=height, enter_editmode=False, location=center)
obj = bpy.context.active_object
# オブジェクト名を設定する
obj.name = cone_name
#√3の過去光円錐 底面を円柱円板で作る
import bpy
import math
import mathutils
kakodoai2radius = 3**(0.5)
cylinder_name = "√3過去 円柱円板" # オブジェクト名
cylinder_radius = kakodoai2radius # 半径
cylinder_height = 0 # 高さ
cylinder_center = mathutils.Vector((0.0, 0.0, -kakodoai2radius)) # 中心位置
bpy.ops.mesh.primitive_cylinder_add(radius=cylinder_radius, depth=cylinder_height, enter_editmode=False, location=cylinder_center)
cyl_obj = bpy.context.active_object
cyl_obj.name = cylinder_name
#2単位の過去光円錐 底面を円柱円板で作る
import bpy
import math
import mathutils
kakodoai2radius = 2
cylinder_name = " 2単位 過去 円柱円板" # オブジェクト名
cylinder_radius = kakodoai2radius # 半径
cylinder_height = 0 # 高さ
cylinder_center = mathutils.Vector((0.0, 0.0, -2)) # 中心位置
bpy.ops.mesh.primitive_cylinder_add(radius=cylinder_radius, depth=cylinder_height, enter_editmode=False, location=cylinder_center)
cyl_obj = bpy.context.active_object
cyl_obj.name = cylinder_name